Thymeleaf的主要目标是将优雅的自然模板带到开发工作流程中,并将HTML在浏览器中正确显示,并且可以作为静态原型,让开发团队能更容易地协作。Thymeleaf能够处理HTML,XML,JavaScript,CSS甚至纯文本。
长期以来,jsp在视图领域有非常重要的地位,随着时间的变迁,出现了以为新的挑战者:Thymeleaf,Thymeleaf是原生的,不依赖标签库,他能够在接收原始HTML的地方进行编译和渲染。因为它没有与Servlet规范耦合,因此Thymeleaf模板能进入jsp所无法涉足的领域。.
1、Thymeleaf基本使用
1.1、创建项目
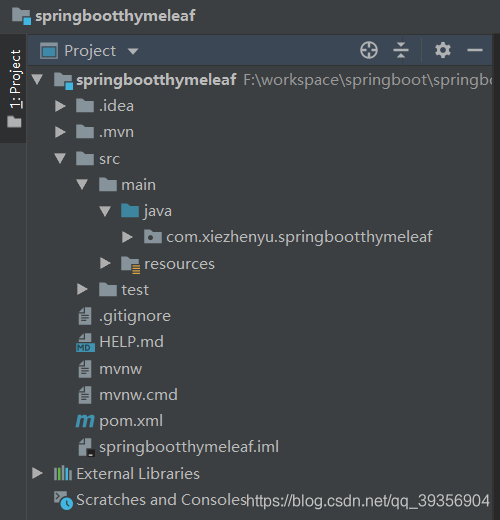
1.2、修改POM文件,添加Thymeleaf依赖
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.6.RELEASE</version> <relativePath/> </parent> <groupId>com.xiezhenyu</groupId> <artifactId>springbootthymeleaf</artifactId> <version>0.0.1-SNAPSHOT</version> <name>springbootthymeleaf</name> <description>Demo project for Spring Boot</description>
<properties> <java.version>1.8</java.version> </properties>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
</project>
|
1.3、创建Controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14
|
@Controller public class PageController {
@GetMapping("/show") public String showPage(Model model){ model.addAttribute("msg","Hello Thymeleaf!"); return "index"; } }
|
1.4、创建视图
1 2 3 4 5 6 7 8 9 10 11
| <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <head> <title>Title</title> </head> <body> <span th:text="谢振瑜"></span> <hr/> <span th:text="${msg}"></span> </body> </html>
|
1.5、运行结果
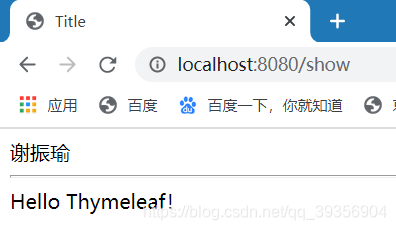
2、Thymeleaf语法
命名空间: xmlns:th=”http://www.thymeleaf.org"
2.1、字符串与变量输出操作
2.1.1、th:text
作用:在页面中输出值。
注意:如果标签中含有内容,它会被th:text标记的内容替换掉。
1 2
| <span th:text="谢振瑜"></span> <span th:text="${msg}"></span>
|
2.1.2、th:value
作用:可以将一个值放入到input标签的value中。
1
| <input th:value="${msg}"/>
|
2.2、字符串操作
Thymeleaf提供了一些内置对象,内置对象可直接在模板中使用。这些对象是以#引用的。
2.2.1、使用内置对象的语法
- 引用内置对象需要使用 #
- 大部分内置对象的名称都以 s 结尾。如:strings、numbers、datas
1
| ${#strings.isEmpty(key)}
|
判断字符串是否为空,如果为空返回true,否则返回false。
1
| <span th:text="${#strings.isEmpty(msg)}"></span>
|
1
| ${#strings.contains(msg,'T')}
|
判断字符串是否包含指定的子串,如果包含返回true,否则返回false。
1
| <span th:text="${#strings.contains(msg,'T')}"></span>
|
1
| ${#strings.startWith(msg,'a')}
|
判断字符串是否以子串开头,如果是返回true,否则返回false。
1
| <span th:text="${#strings.startWith(msg,'a')}"></span>
|
1
| ${#strings.endWith(msg,'a')}
|
判断字符串是否以子串结尾,如果是返回true,否则返回false。
1
| <span th:text="${#strings.endWith(msg,'a')}"></span>
|
返回字符串的长度。
1
| <span th:text="${#strings.length(msg)}"></span>
|
1
| ${#strings.indexOf(msg,'a')}
|
查找子串的位置,并返回子串的下标,如果没有找到则返回-1。
1
| <span th:text="${#strings.indexOf(msg,'a')}"></span>
|
1 2
| ${#strings.substring(msg,2)} ${#strings.substring(msg,2,5)}
|
截取子串,用法与jdk String类下 SubString方法相同。
1
| <span th:text="${#strings.substring(msg,2,5)}"></span>
|
1 2
| ${#strings.toUpperCase(msg)} ${#strings.toLowerCase(msg)}
|
字符串大小写转换。
1
| <span th:text="${#strings.toLowerCase(msg)}"></span>
|
2.3、日期格式化处理
格式化日期,默认的以浏览器默认语言为格式标准。
1
| ${#dates.format(key,'yyy/MM/dd')}
|
按自定义的格式做日期转换
1 2 3
| ${#dates.yrear(key)} ${#dates.month(key)} ${#dates.day(key)}
|
year:取年 month:取月 day:取日
2.4、条件判断
2.4.1、th:if
作用:条件判断
1 2 3 4 5 6 7 8
| <div> <span th:if="${sex} == '男'"> 性别:男 </span> <span th:if="${sex} == '女'"> 性别:女 </span> </div>
|
2.4.2、th:switch / th:case
作用:
th:switch / th:case 与 java 中的 switch 语句等效,有条件地显示匹配内容。如果有多个匹配结果只选择第一个显示。
th:case=”*” 表示 Java 中 switch 的 default,即没有 case 的值为true时则显示th:case=”*”的内容。
1 2 3 4 5 6 7 8
| <div th:switch="${id}"> <span th:case="1">id:1</span> <span th:case="2">id:2</span> <span th:case="3">id:3</span> <span th:case="4">id:4</span> <span th:case="5">id:5</span> <span th:case="*">id:*</span> </div>
|
2.5、迭代遍历
2.5.1、th:each
作用:迭代器,用于循环迭代集合。
1 2 3 4 5 6 7 8 9 10 11 12
| <table border="1" width="50%"> <tr> <th>ID</th> <th>NAME</th> <th>AGE</th> </tr> <tr th:each="u : ${list}"> <td th:text="${u.id}"></td> <td th:text="${u.name}"></td> <td th:text="${u.age}"></td> </tr> </table>
|
2.5.2、th:each 状态变化
- index: 当前迭代器的索引 从0开始
- count: 当前迭代器对象的计数 从1开始
- size: 被迭代器对象的长度
- odd/even: 布尔值,当前循环是否是奇数/偶数 从0开始
- first: 布尔值,当前循环的是否是第一条,如果是返回true否则返回false
- last: 布尔值,当前循环是否是最后一条,如果是返回true否则返回false
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <table border="1" width="50%"> <tr> <th>ID</th> <th>NAME</th> <th>AGE</th> <th>Index</th> <th>Count</th> <th>Size</th> <th>Odd</th> <th>Even</th> <th>First</th> <th>Last</th> </tr> <tr th:each="u,suibian : ${list}"> <td th:text="${u.id}"></td> <td th:text="${u.name}"></td> <td th:text="${u.age}"></td> <td th:text="${suibian.index}"></td> <td th:text="${suibian.count}"></td> <td th:text="${suibian.size}"></td> <td th:text="${suibian.odd}"></td> <td th:text="${suibian.even}"></td> <td th:text="${suibian.first}"></td> <td th:text="${suibian.last}"></td> </tr> </table>
|
结果:

2.6、th:each 迭代 Map
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <table border="1" width="50%"> <tr> <th>ID</th> <th>NAME</th> <th>AGE</th> <th>Key</th> </tr> <tr th:each="m : ${map}"> <td th:text="${m.value.id}"></td> <td th:text="${m.value.name}"></td> <td th:text="${m.value.age}"></td> <td th:text="${m.key}"></td> </tr> </table>
|
2.7、操作域对象
HttpServletRequest
HttpSession
ServletContext
1 2 3
| request.setAttribute("req","HttpServletRequest"); request.getSession().setAttribute("ses","HttpSession"); request.getSession().getServletContext().setAttribute("app","application");
|
1 2 3 4 5 6
| HttpServletRequest方式一:<span th:text="${#request.getAttribute('req')}"></span><br/> HttpServletRequest方式二:<span th:text="${#httpServletRequest.getAttribute('req')}"></span><br/> HttpSession方式一:<span th:text="${#session.getAttribute('ses')}"></span><br/> HttpSession方式二:<span th:text="${session.ses}"></span><br/> Application方式一::<span th:text="${#session.getServletContext().getAttribute('app')}"></span><br/> Application方式二:<span th:text="${application.app}"></span>
|
2.8、URL表达式
2.8.1、语法
在Thymeleaf中URL表达式的语法格式为@{}
2.8.2、URL类型
绝对路径
1
| <a th:href="@{http://baidu.com}">绝对路径</a>
|
相对路径
1 2 3 4
| <a th:href="@{/show}">相对路径</a>
<a th:href="@{~/project2/resourcename}">相对于服务器的根</a>
|
2.8.3、在URL中传递参数
在普通格式的URL中传递参数
1 2 3 4
| <a th:href="@{/show?id=1&name=zhansan}">普通URL格式传参</a> <a th:href="@{/show(id=1,name=zhansan)}">普通URL格式传参</a> <a th:href="@{/'show?id=' + ${id} + '&name=' + ${name}}">普通URL格式传参</a> <a th:href="@{/show(id=${id},name=${name})}">普通URL格式传参</a>
|
在restful格式的URL中传递参数
1 2 3 4
| <a th:href="@{/show/{id}(id=1)}">restful格式传参</a> <a th:href="@{/show/{id}/{name}(id=1,name=admin)}">restful格式传参</a> <a th:href="@{/show/{id}(id=1,name=admin)}">restful格式传参</a> <a th:href="@{/show/{id}(id=${id},name=${name})}">restful格式传参</a>
|
2.9、在配置文件中配置Thymeleaf
1 2 3 4 5 6 7 8 9 10 11 12
| #默认路径 spring.thymeleaf.prefix=classpath:/templates/suibian/ #默认扩展名 spring.thymeleaf.suffix=.html #配置视图模板类型,HTML4、HTML5,(如果视图模板使用的html5则需要配置) spring.thymeleaf.mode=HTML #配置thymeleaf引擎的编码 spring.thymeleaf.encoding=UTF-8 #配置相应类型 spring.thymeleaf.servlet.content-type=text/html #配置thymeleaf的页面缓存,true为缓存,false为不缓存 spring.thymeleaf.cache=false
|